🎈 Before getting to width and height, we need to have something to give a width and height to.
🎈 For this purpose, you are going to be introduced to a new HTML element known as “div”.
🎈 We don't need much complication so
you can define 'div' as a flexible box or a container. What we mean by that is, it can be a paragraph holder, header holder, it can be a box, a circle, a rectangle, a square, anything you want.
🎈 In this width and height session
you are going to learn how to create a rectangle.
Follow along
1.Write your basic html code.
<html>
<head>
<title>Rectangle</title>
</head>
<body>
</body>
</html>
2.Inside the body tag open a 'div' tag which looks like this one <div></div>
<body>
<div></div>
</body>
⭐ Now if you go and open your file after saving it, you'll find out that nothing will appear on your webpage. This is because we haven't yet defined what to do with our div tag. There is no way the webpage will know what you want to do with your div without
telling it. 👦👦
⭐ In the process, have you wondered how you can give your div a certain width and height? Well, that is the work of CSS.
⭐ There are ways we can code on CSS to style up our webpage but for
now we are going to style up our webpage through “Internal CSS”.
⭐ Internal CSS is a way in which you can have your CSS code inside the same file. You can have your internal CSS by going to the head tag and adding style tags (<style></style>)
inside it.
<html>
<head>
<title>Rectangle</title>
<style></style>
</head>
<body>
<div></div>
</body>
</html>
3.Inside the style tag write div and open curly brackets.
<style>
div {
}
</style>
4.Inside the curly brackets, you are going to write the code below.
But before that, you need to understand what it means. The entitles like width, height, color, font-size and so on are known as
Properties. And the entitles which consist of the adjustment you need like 100px, red, blur, etc… are known as
Values.
Measurements can be in pixels, percents, vh and vw.
⭐ When writing a CSS code, you should separate properties and values with a colon(:) and add semicolon at the end of the value(;).
This format of CSS code
is known as the “property value pair”. For example “width: 100px;” this is called a property value pair.
<style>
div {
width: 500px;
height:
500px;
}
</style>
5.Add a background color inside the curly brackets you already created. It makes the div to appear on your webpage.
<style>
div {
width: 500px;
height:
500px;
background-color: blue;
}
</style>
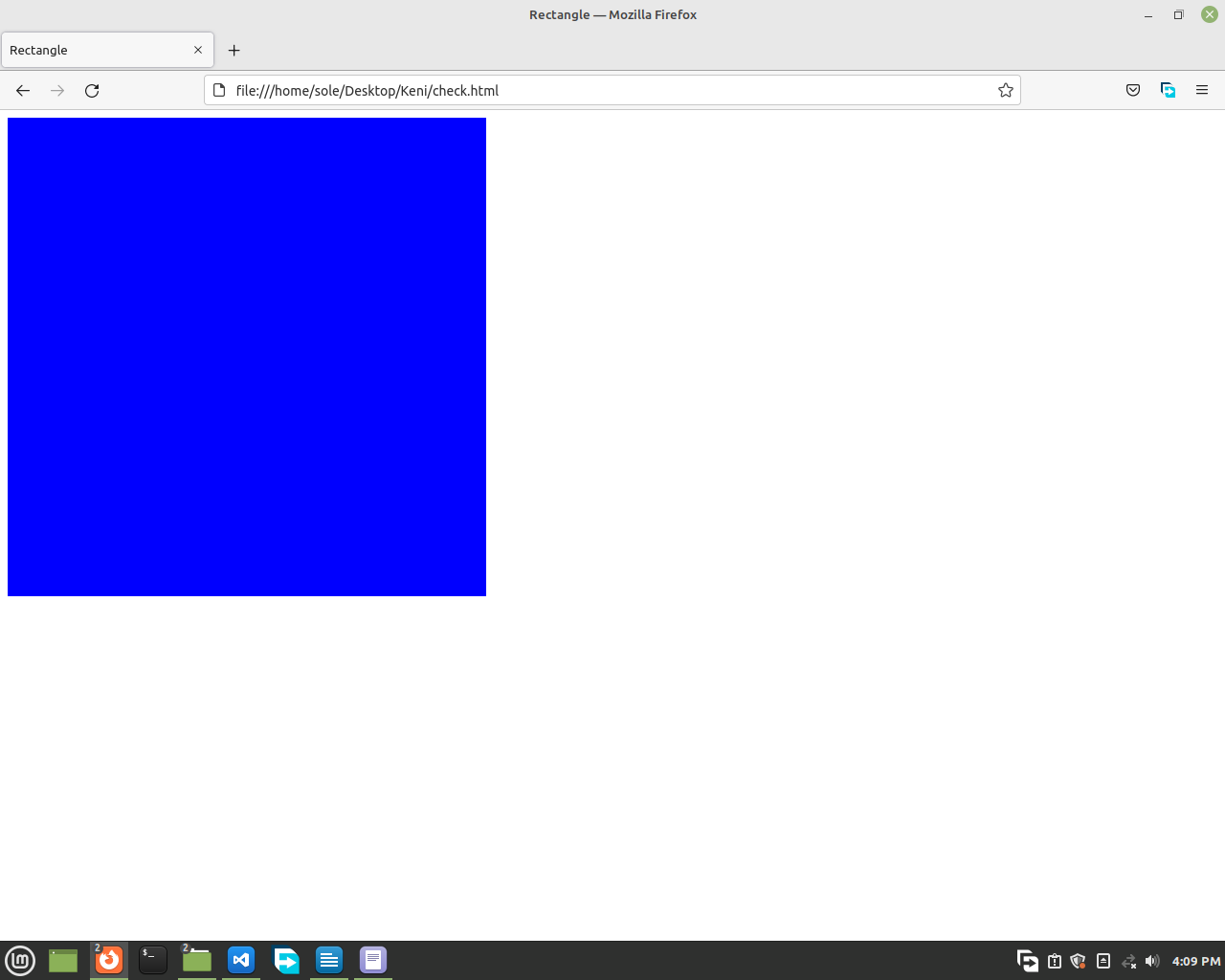
🕵But if you are wondering about why it doesn't appear on your webpage without adjusting a background color, it's because any div has a default background color of transparent.