π If you see something new on the code, feel free you will learn everything later. For now just try to concentrate on
.bod2's position property.
β Position has 5 main values. They are static, relative, absolute, sticky, fixed.
2.11.1. Static
β Static is the
default value given to an element in CSS.
Full code:
<html>
<head>
<style>
* {
margin: 0;
padding:
0;
box-sizing: border-box;
}
body {
display: flex;
width:
100vw;
height: 100vh;
}
.bod {
width: 200px;
height:
200px;
background-color: rgb(128, 95, 95);
}
.bod2 {
position: static;
top:
100px;
left: 100px;
width: 200px;
height: 200px;
background-color:
rgb(201, 186, 186);
}
.bod3 {
width: 200px;
height:
200px;
background-color: rgb(175, 6, 6);
}
</style>
</head>
<body>
<div class="bod"></div>
<div class="bod2"></div>
<div
class="bod3"></div>
</body>
</html>
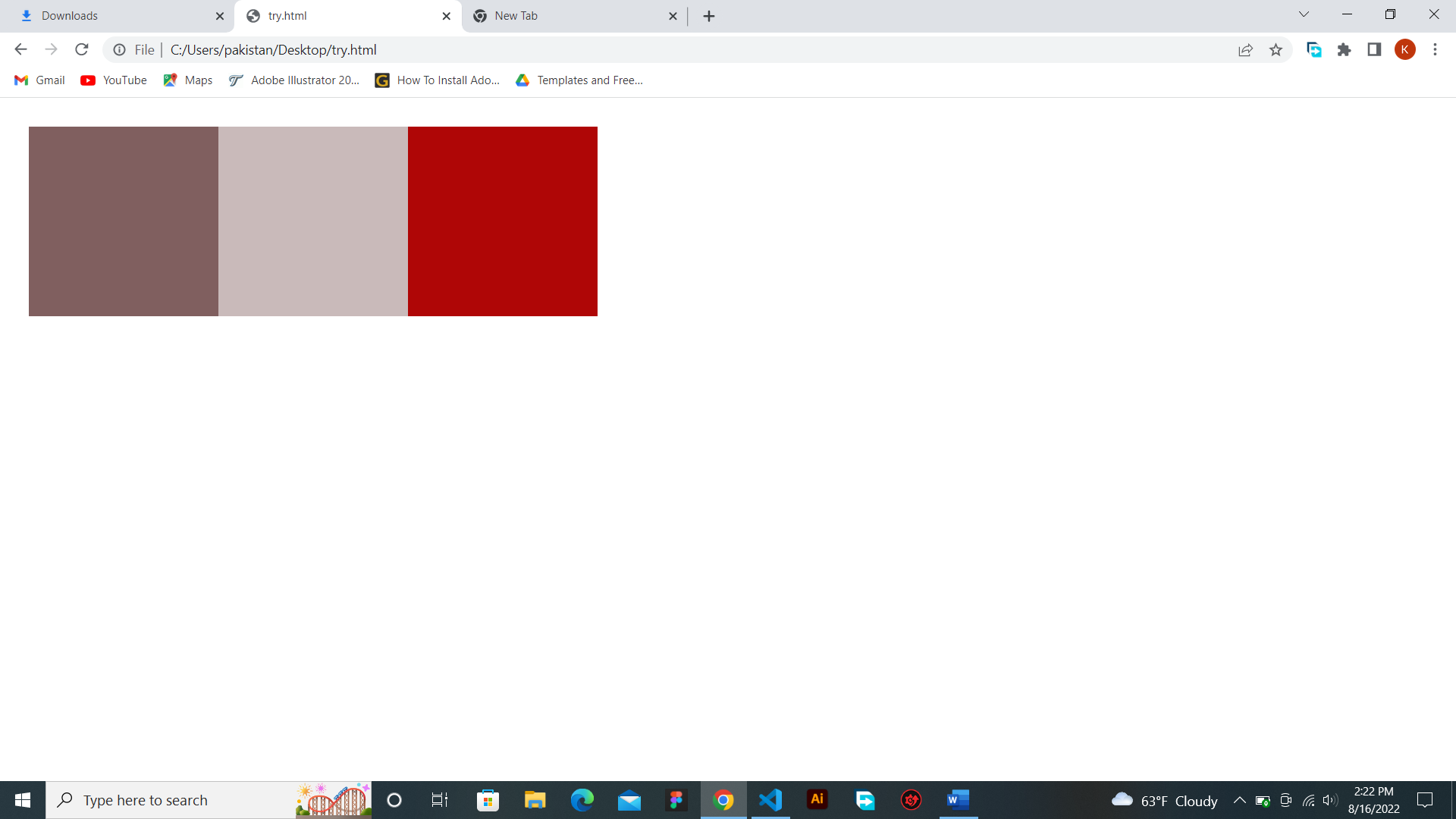
2.11.2. Relative
When we give an element a value of relative, it makes the element to flow wherever it wants and also the first space given to it is not removed.
Full code:
<html>
<head>
<style>
* {
margin: 0;
padding:
0;
box-sizing: border-box;
}
body {
display: flex;
width:
100vw;
height: 100vh;
}
.bod {
width: 200px;
height:
200px;
background-color: rgb(128, 95, 95);
}
.bod2 {
position: relative;
top:
100px;
left: 100px;
width: 200px;
height: 200px;
background-color:
rgb(201, 186, 186);
}
.bod3 {
width: 200px;
height:
200px;
background-color: rgb(175, 6, 6);
}
</style>
<head>
<body>
<div class="bod"></div>
<div class="bod2"></div>
<div
class="bod3"></div>
</body>
</html>
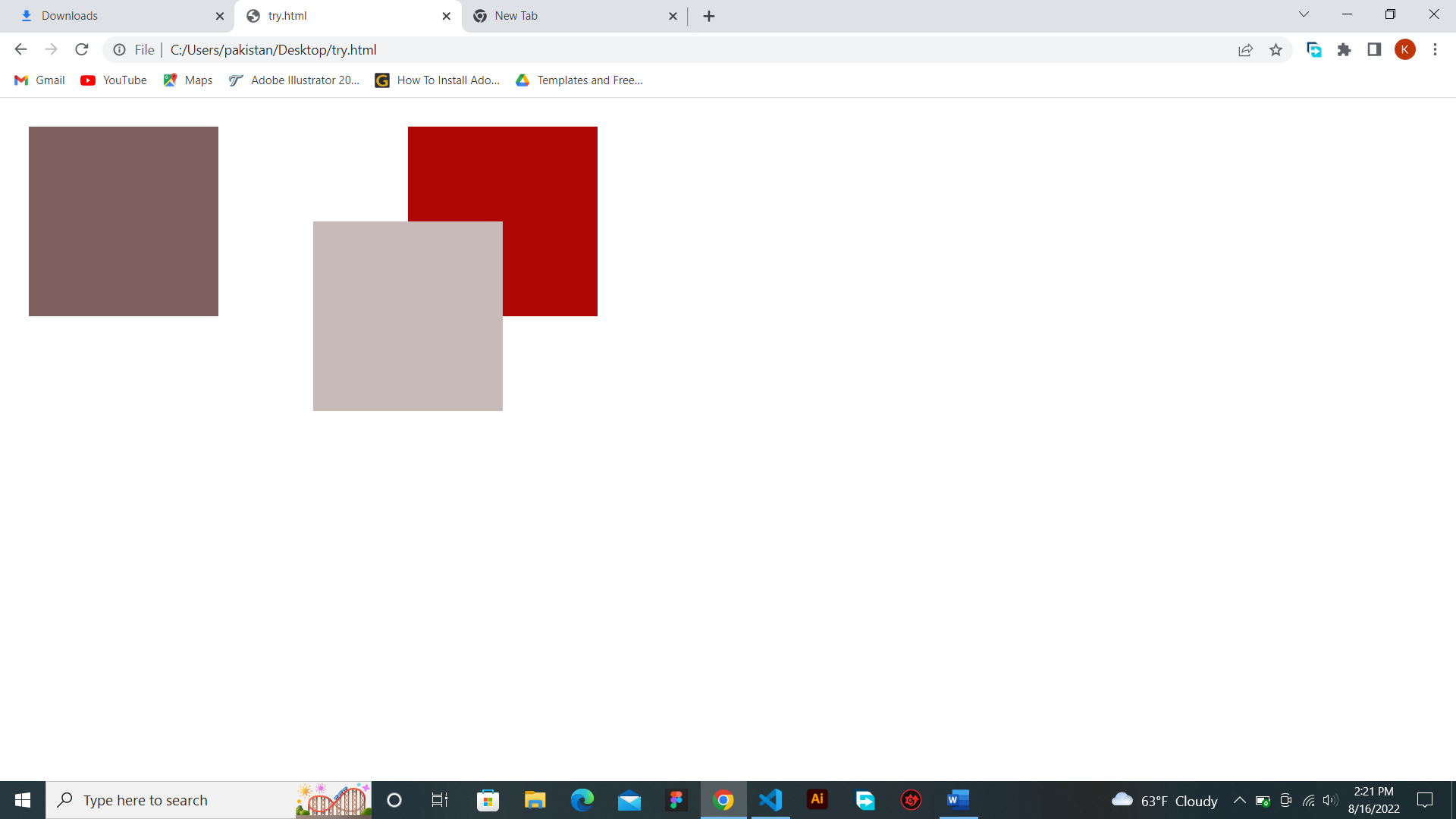
2.11.3. Absolute
It's just like relative but, if you don't want the space you had before this removes it. Compare the last image (relative) with the next image (absolute).
Full code:
<html>
<head>
<style>
* {
margin: 0;
padding:
0;
box-sizing: border-box;
}
body {
display: flex;
width:
100vw;
height: 100vh;
}
.bod {
width: 200px;
height:
200px;
background-color: rgb(128, 95, 95);
}
.bod2 {
position: absolute;
top:
100px;
left: 100px;
width: 200px;
height: 200px;
background-color:
rgb(201, 186, 186);
}
.bod3 {
width: 200px;
height:
200px;
background-color: rgb(175, 6, 6);
}
</style>
</head>
<body>
<div class="bod"></div>
<div class="bod2"></div>
<div
class="bod3"></div>
</body>
</html>
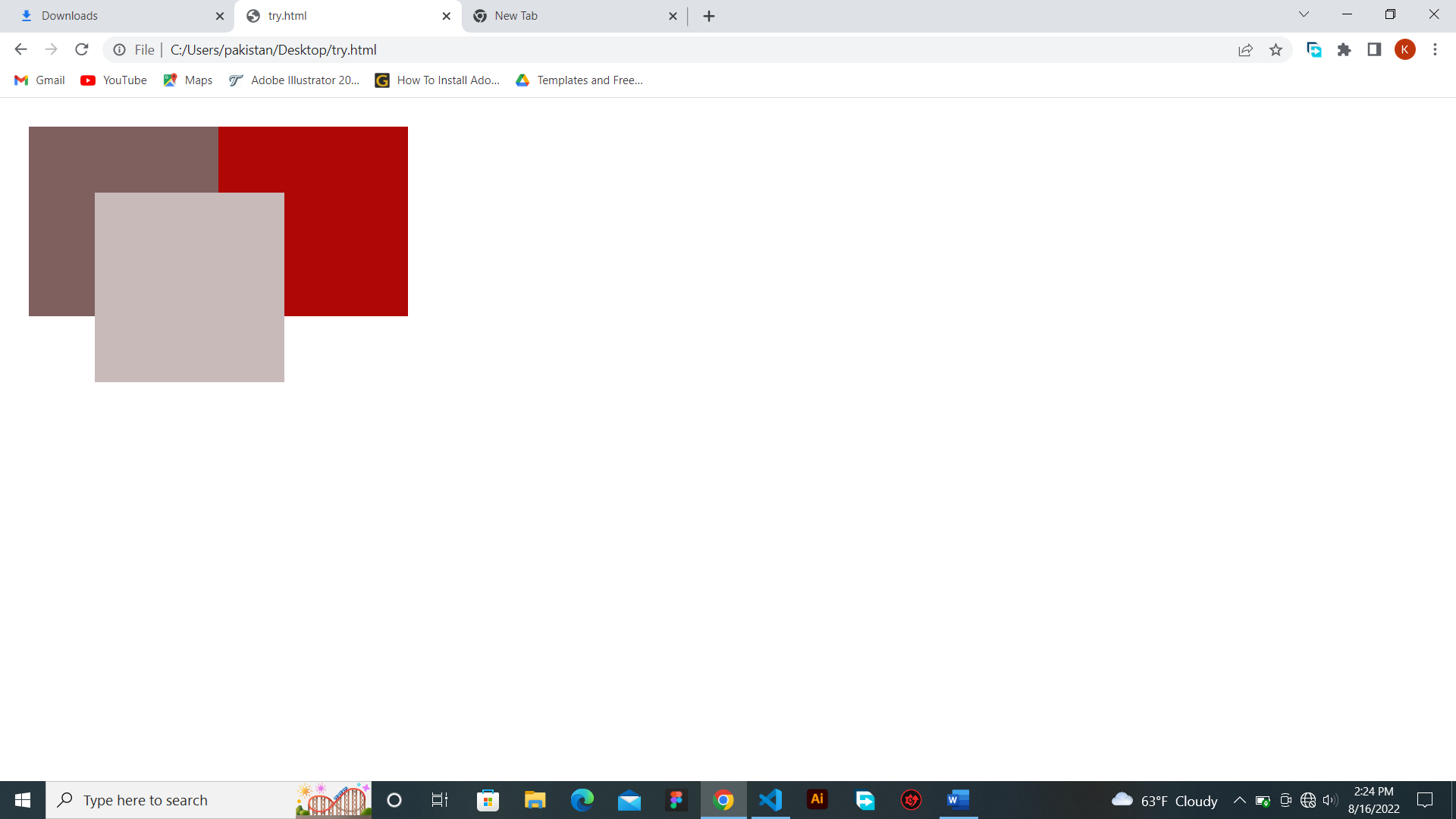
2.11.4. Sticky
β Sticky sticks the element when you scroll over it. It won't remove the previous space it had. To understand try the full code here.
Full code:
<html>
<head>
<style>
* {
margin: 0;
padding:
0;
box-sizing: border-box;
}
body {
display: flex;
align-items:
center;
justify-content: center;
width:
99vw;
height: 200vh;
}
.bod {
width: 200px;
height:
200px;
background-color: rgb(128, 95, 95);
}
.bod2 {
position: sticky;
top:
100px;
left: 100px;
width: 200px;
height: 200px;
background-color:
rgb(201, 186, 186);
}
.bod3 {
width: 200px;
height:
200px;
background-color: rgb(175, 6, 6);
}
</style>
</head>
<body>
<div class="bod"></div>
<div class="bod2"></div>
<div
class="bod3"></div>
</body>
</html>
β I hope you see a difference in this code from other previous codes, not the position but I changed the width and height of the body. Width to 99vw and Height to 200vh.
β The width was 100vw on previous ones, but now we will change it to 99vw, because if the height is 200vh, there is a vertical scrollbar (top to bottom). And if we make our width 100vw, the width will also include the vertical
scrollbar's width and that will create a horizontal scrollbar (left to right), which is so annoying and not needed. So, we will just make our width 99vw or a little lower.
ββ Try to look it yourself by making the width of the body 100vw and the height of the body 200vh.
2.11.5. Fixed
β Fixed sticks the element when you scroll over it. It will remove the previous space it had.
Full code:
<html>
<head>
<style>
* {
margin: 0;
padding:
0;
box-sizing: border-box;
}
body {
display: flex;
align-items:
center;
justify-content: center;
width:
99vw;
height: 200vh;
}
.bod {
width: 200px;
height:
200px;
background-color: rgb(128, 95, 95);
}
.bod2 {
position: fixed;
top:
100px;
left: 100px;
width: 200px;
height: 200px;
background-color:
rgb(201, 186, 186);
}
.bod3 {
width: 200px;
height:
200px;
background-color: rgb(175, 6, 6);
}
</style>
</head>
<body>
<div class="bod"></div>
<div class="bod2"></div>
<div
class="bod3"></div>
</body>
</html>
β
Task: See the other difference of sticky and fixed by running the code of both of them. And when you find the difference, go to your next lesson. Don't just read it ok, Run itπ! Run it!