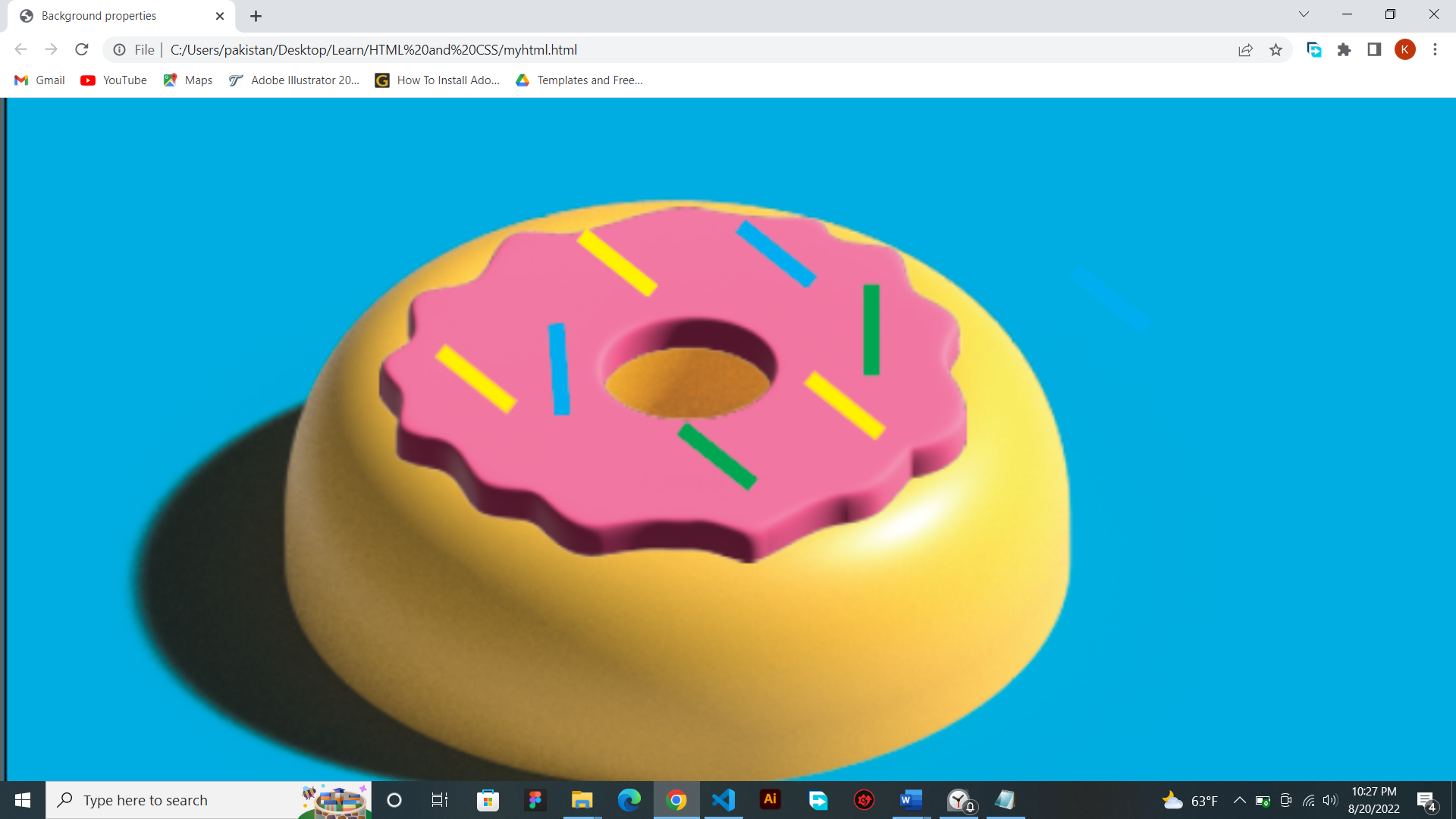
In the webpage above, you can see the body has a background of a donut image. In this session, we are going to create a webpage with a background image, and some adjustments.
1.
Write the basic html code.
<html>
<head>
<title>Background properties</title>
</head>
<body>
</body>
</html>
2. Open a style tag in the head tag for later use to style your div.
<head>
<title>Background properties</title>
<style>
</style>
</head>
3. Go to the style tag and write 'body' followed by curly brackets.
<head>
<title>Background properties</title>
<style>
body {
}
</style>
</head>
4. Inside the curly brackets write background image with the image path in a url with brackets. Use an image you want to use. You can use an image you found either on your computer or online, url works for both.
π Add a width
and height of 400% to your body tag too
<head>
<title>Background properties</title>
<style>
body {
width:400%;
height: 400%;
background-image:
url(C:/Users/pakistan/Pictures/Screenshots/donut.png);
}
</style>
</head>
5.Save your file and open it on your browser.
π You can see that the image repeats itself both on the x and y axis.
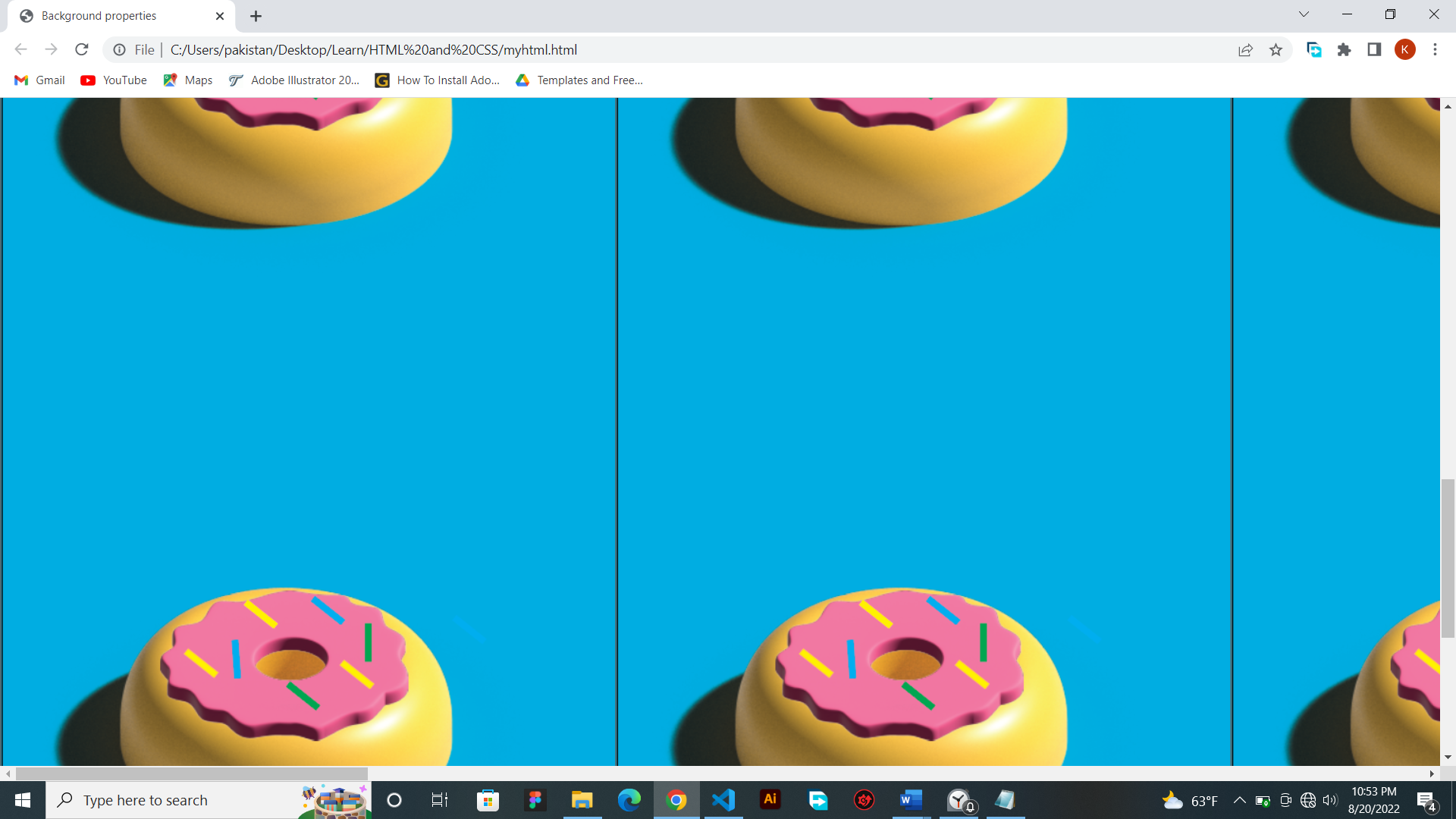
2.3.1. Background Repeat
π It enables you to adjust the repetition of your background.
π You can set the property to a value of 'none' which stops background image from repeating itself over and over.
You can also
set the property to 'repeat-x' which makes the background to repeat itself only in the x axis while 'repeat-y' makes the background repeat itself only in the y axis.
π background-repeat: repeat-x;
<head>
<title>Background properties</title>
<style>
body {
background-repeat: repeat-x;
width:400%;
height: 400%;
background-image:
url(C:/Users/pakistan/Pictures/Screenshots/donut.png);
}
</style>
</head>
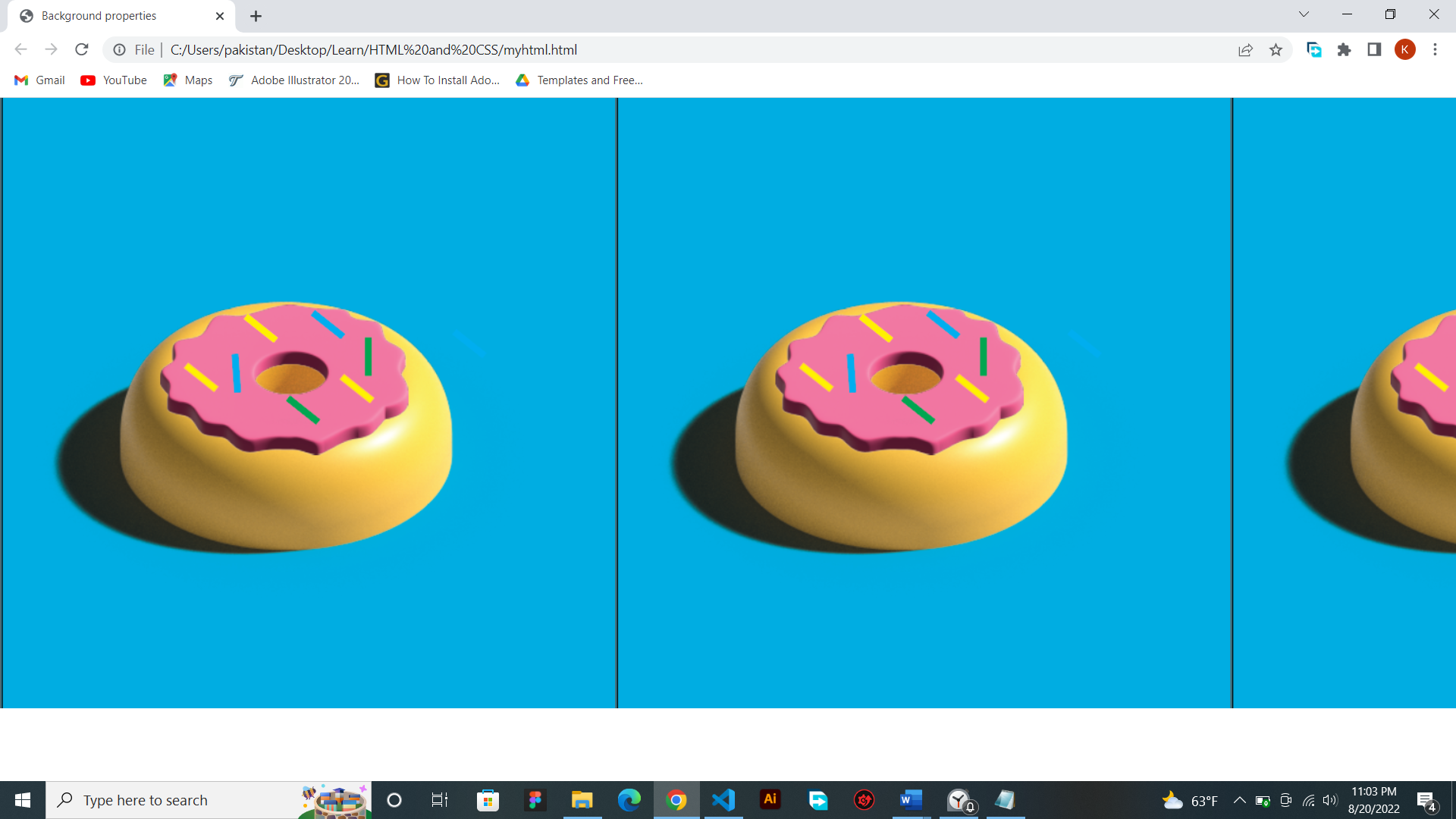
<head>
<title>Background properties</title>
<style>
body {
background-repeat: repeat-y;
width:400%;
height: 400%;
background-image:
url(C:/Users/pakistan/Pictures/Screenshots/donut.png);
}
</style>
</head>
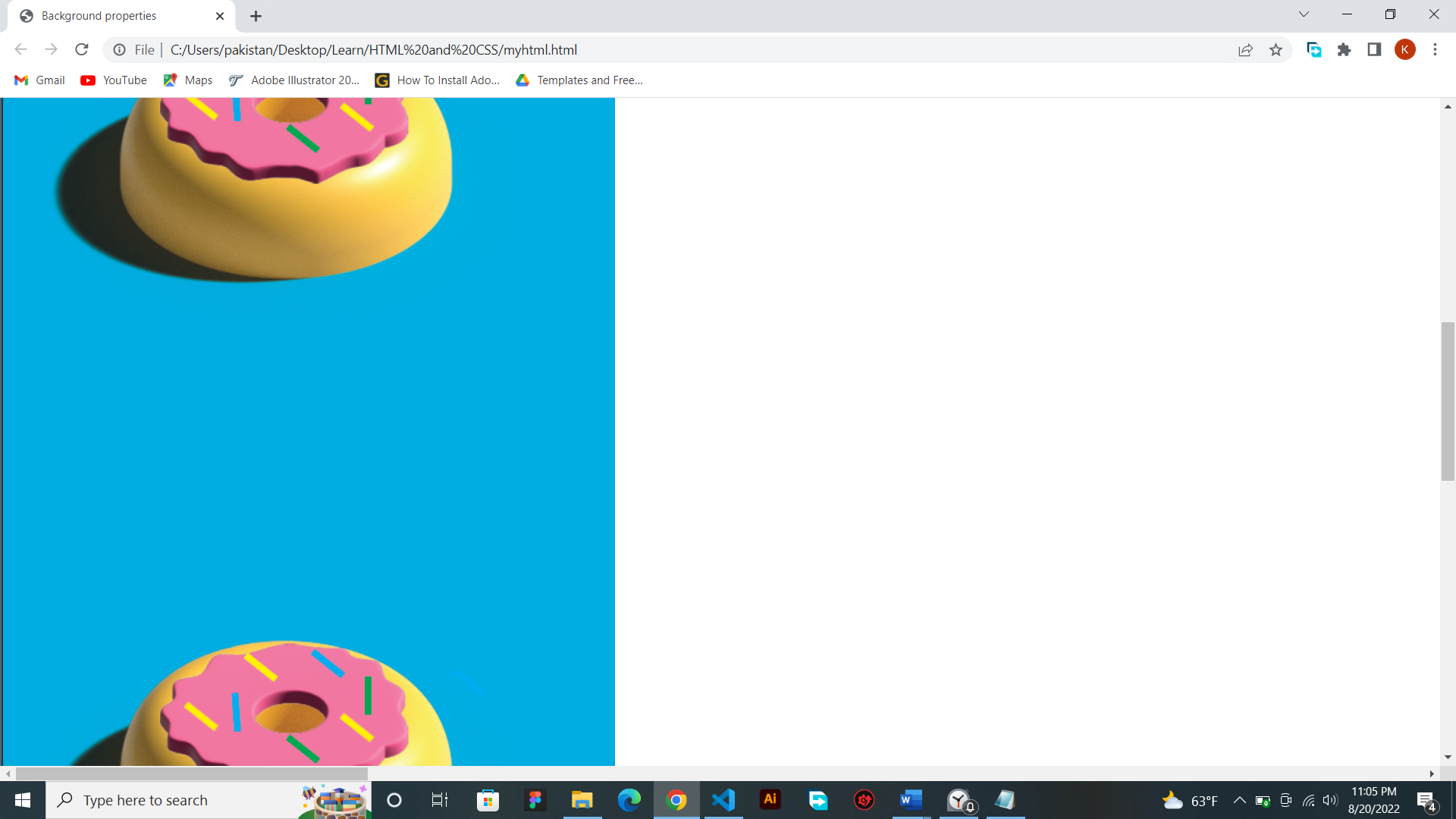
π We made you adjust your width and height property to a value of 400%, to demonstrate how the background works in the x and y axis.
π The βbackground-repeat: no-repeatβ key value pair
will be hard to be demonstrated in the width and height of 400%;
π So go to your styles tag and remove the width and height properties from the body,
<head>
<title>Background properties</title>
<style>
body {
background-repeat: no-repeat;
background-image:
url(C:/Users/pakistan/Pictures/Screenshots/donut.png);
}
</style>
</head>
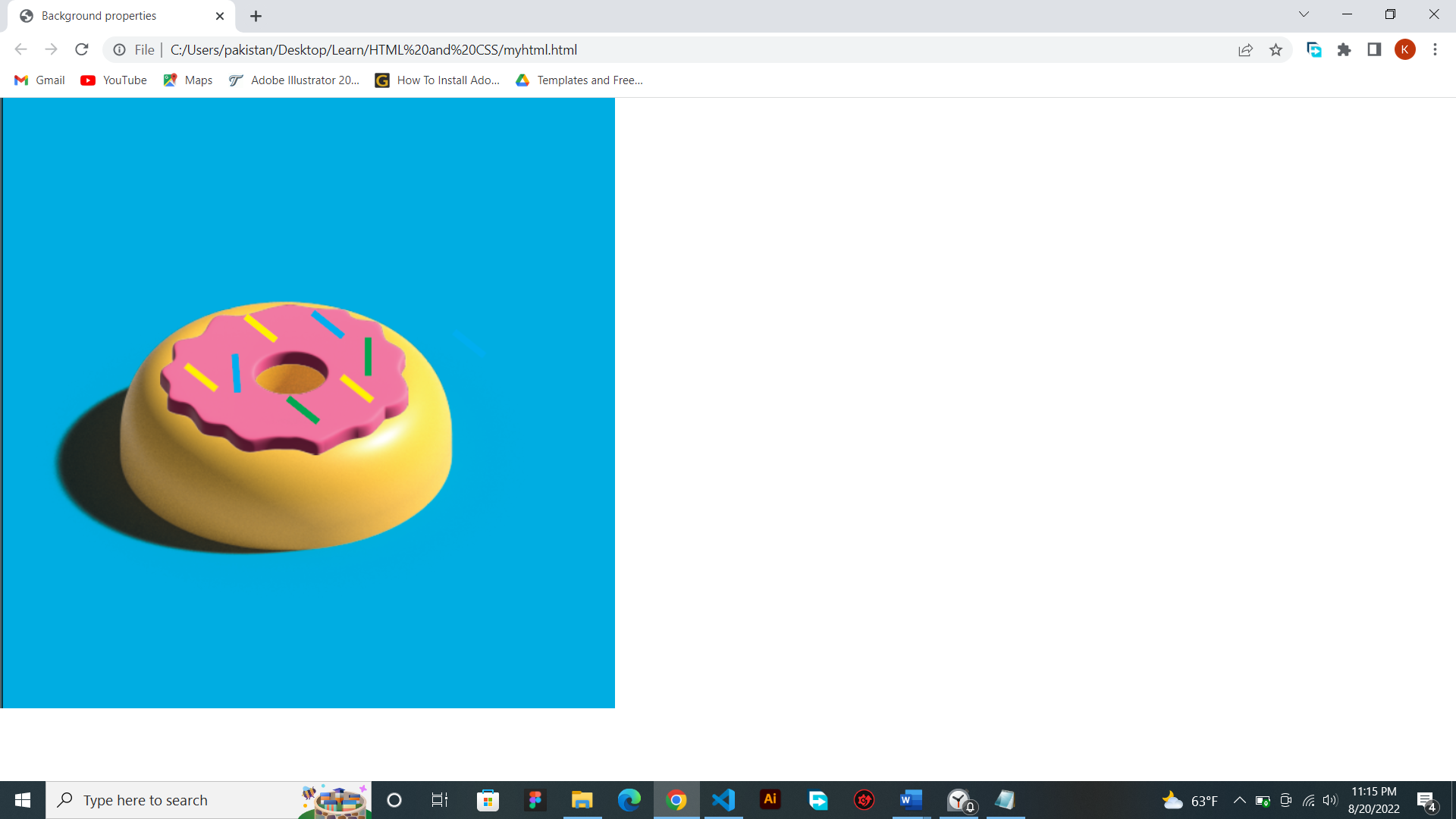
π As you can see only a single image appears when you give it the value of no-repeat.
2.3.2. Background Position
π If you want to align your background image to the center,at the left right or something else you can use background position. The values are: top, left, bottom and center
<head>
<title>Background properties</title>
<style>
body {
background-position: center;
background-repeat:
no-repeat;
background-image: url(C:/Users/pakistan/Pictures/Screenshots/donut.png);
}
</style>
</head>
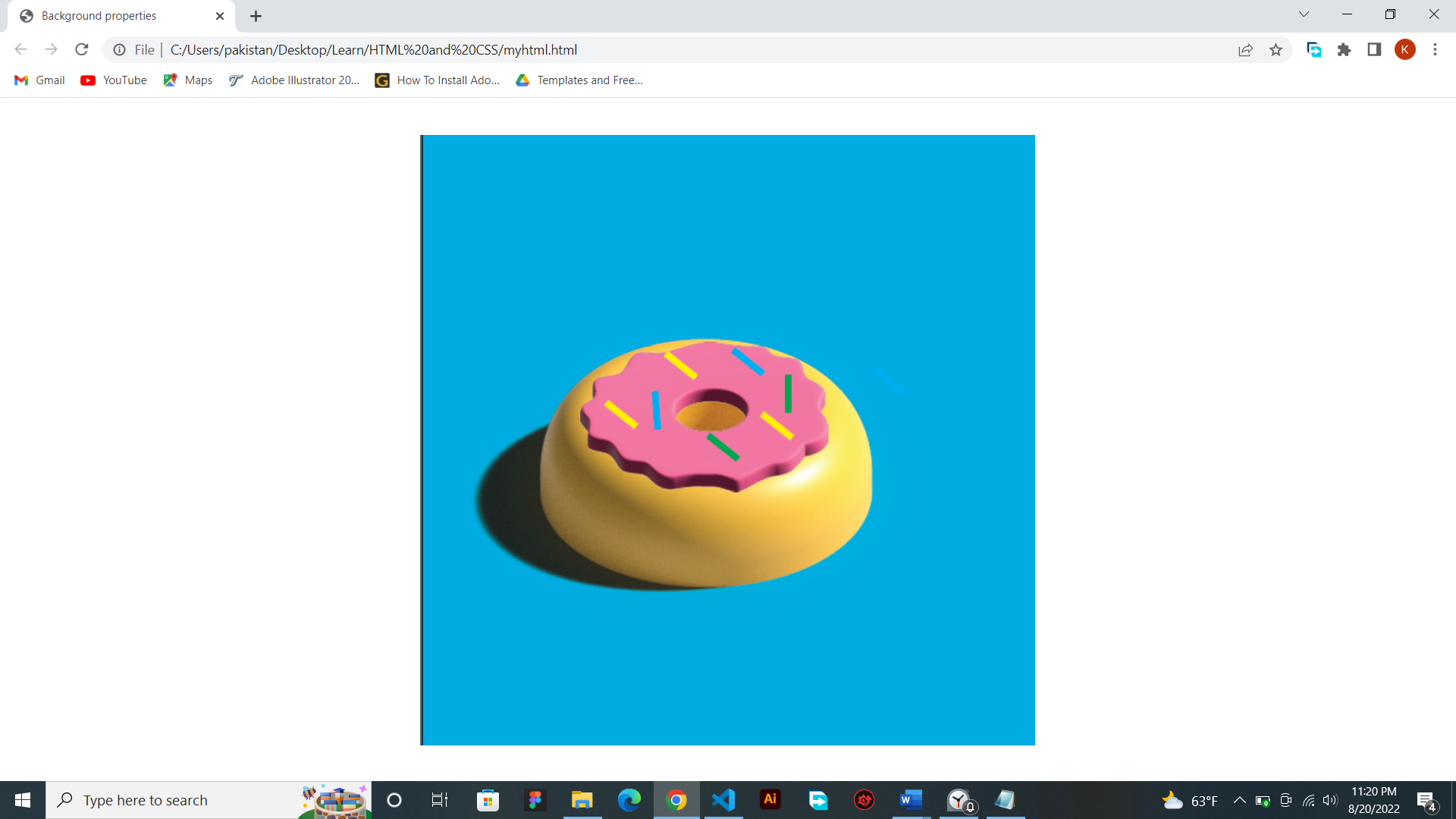
2.3.3. Background Attachment
π If you want your background to never move when you scroll you can use a value called 'fixed'. There is another default value called scroll which just goes with the scroll.
background-attachment:
fixed;
π You will sometimes use this property when working on scrollable webpages.
2.3.4. Background size
π If you have a big image that doesn't fit in your div, or a smaller image that doesn't fit in your div, you can make the image cover your div by using background-size.
background-size: cover;
background-size:
contain;
Cover fully covers the div with the image.
Contain makes the image scaled as large as possible to fit in the div.
<head>
<title>Background properties</title>
<style>
body {
background-size: cover;
background-position:
center;
background-repeat: no-repeat;
background-image:url(C:/Users/pakistan/Pictures/Screenshots/donut.png);
}
</style>
</head>