nth-child is used to select the child elements of a parent.
To make it clear lets take this example.
<div class="parentDiv">
<p class="par">Paragraph1</p>
<p class="par">Paragraph2</p>
<p class="par">Paragraph3</p>
<p class="par">Paragraph4</p>
</div>
As you can see from above the parentDiv has 4 children.
Let's say you want to change the color of Paragraph2, the easy way to do that is to use the "nth-child" selector.
To do this we should have a class that is
common for all the 4 children. In our case "par".
<style>
.par:nth-child(2) {
color: orange;
}
</style>
This changes the color of Paragraph2 to orange.
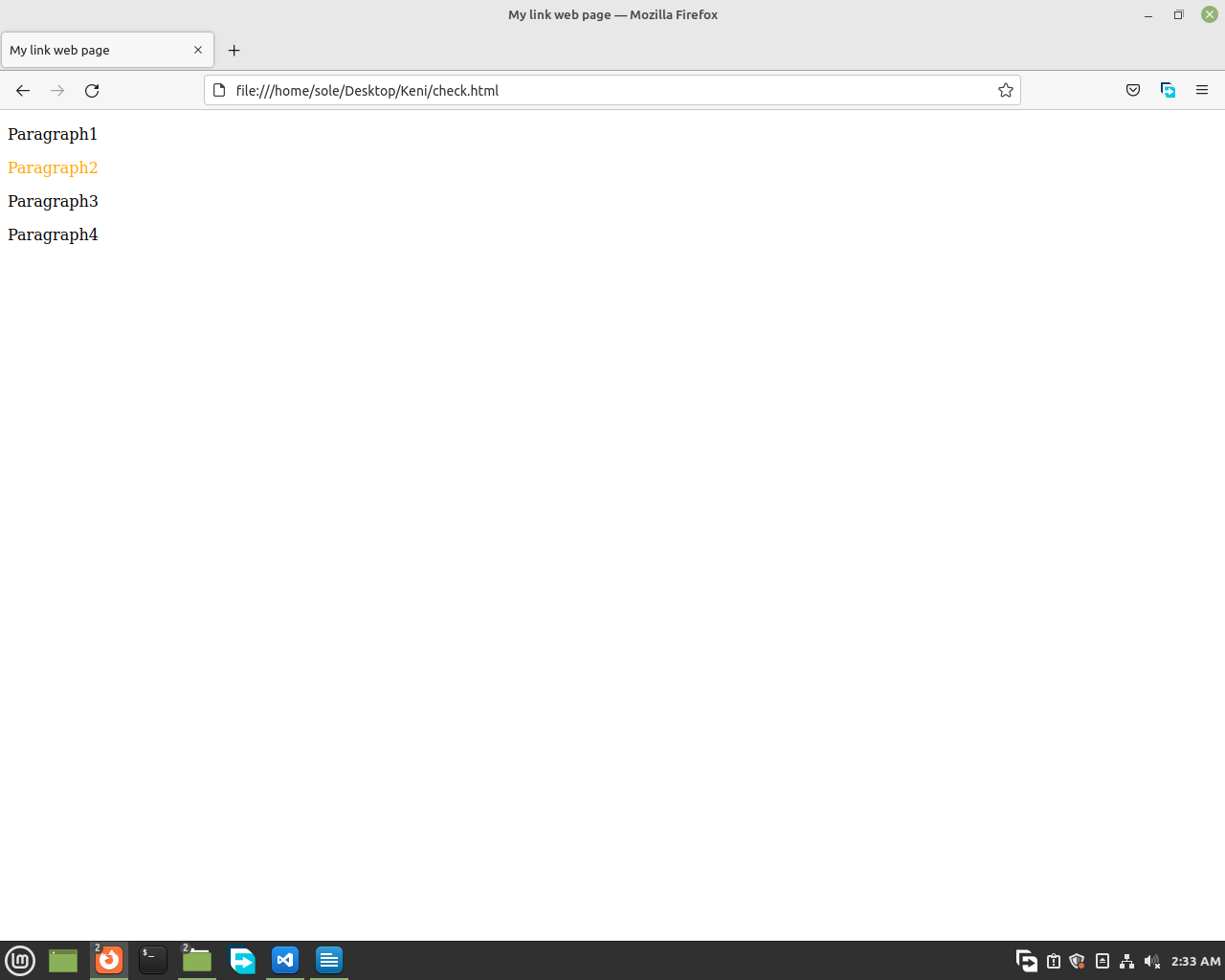
Example2
Let's take another example to learn more about nth-child.
<div class="parentDiv">
<h1>New Child </h1>
<p class="par">Paragraph1</p>
<p class="par">Paragraph2</p>
<p class="par">Paragraph3</p>
<p class="par">Paragraph4</p>
</div>
If you write the same css code we wrote in our first example what do you think will happen??
<style>
.par:nth-child(2) {
color: orange;
}
</style>
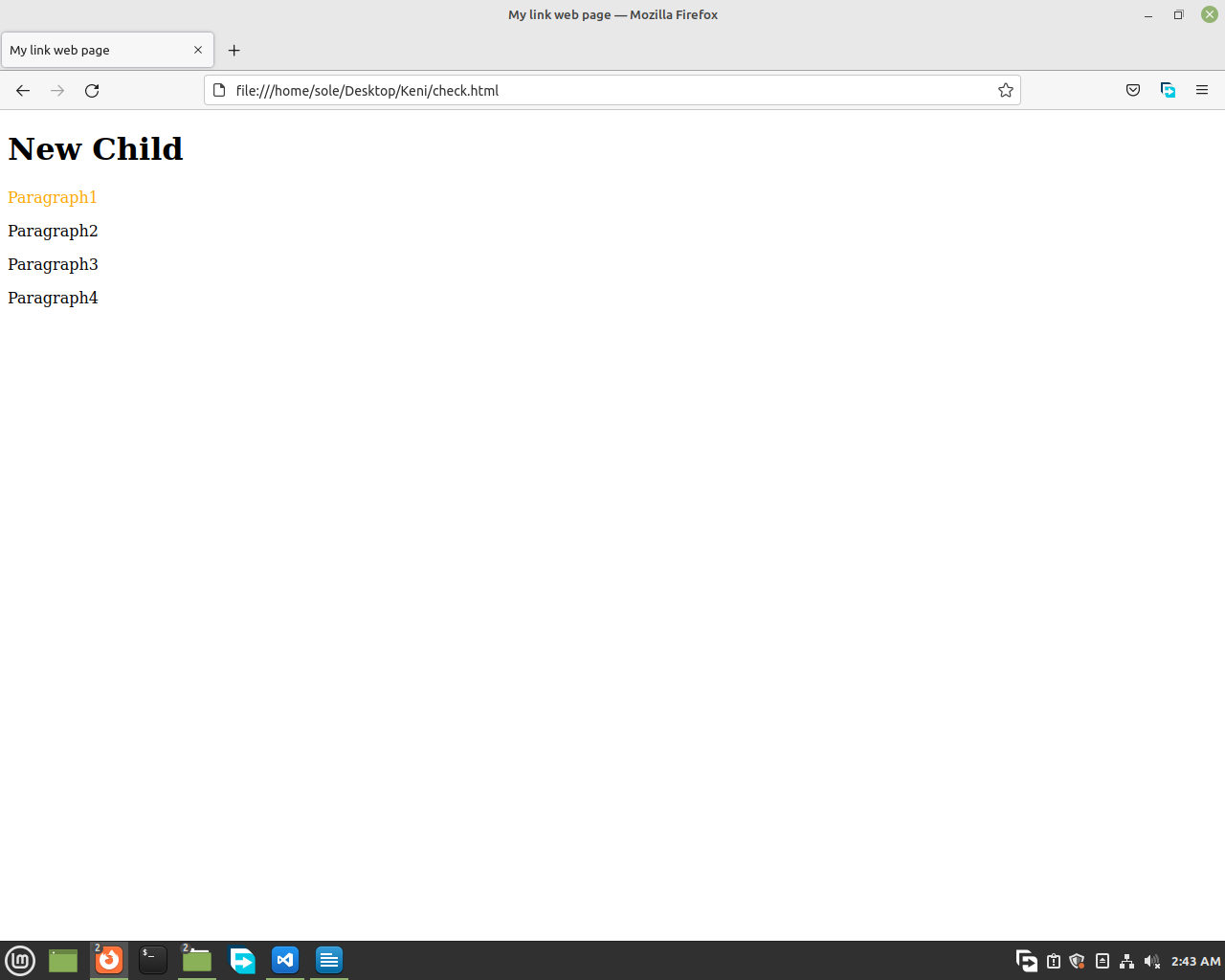
Yes! Paragraph1 is going to change its color to orange. Why? Good Question.
First off let's take this line of code to further understand the topic.
.par:nth-child(2) means
🙂 First it finds elements with a class "par" and then goes to the parent. Then it will select its 2nd child.
⭐ So in example two the 2nd child is paragraph1 that's why its color changed.
Example3.
<div class="parentDiv">
<h1>New Child </h1>
<h2> New Child 2</h1>
<p class="par">Paragraph1</p>
<p
class="par">Paragraph2</p>
<p class="par">Paragraph3</p>
<p class="par">Paragraph4</p>
</div>
<style>
.par:nth-child(2) {
color: orange;
}
</style>
What do you think will happen?
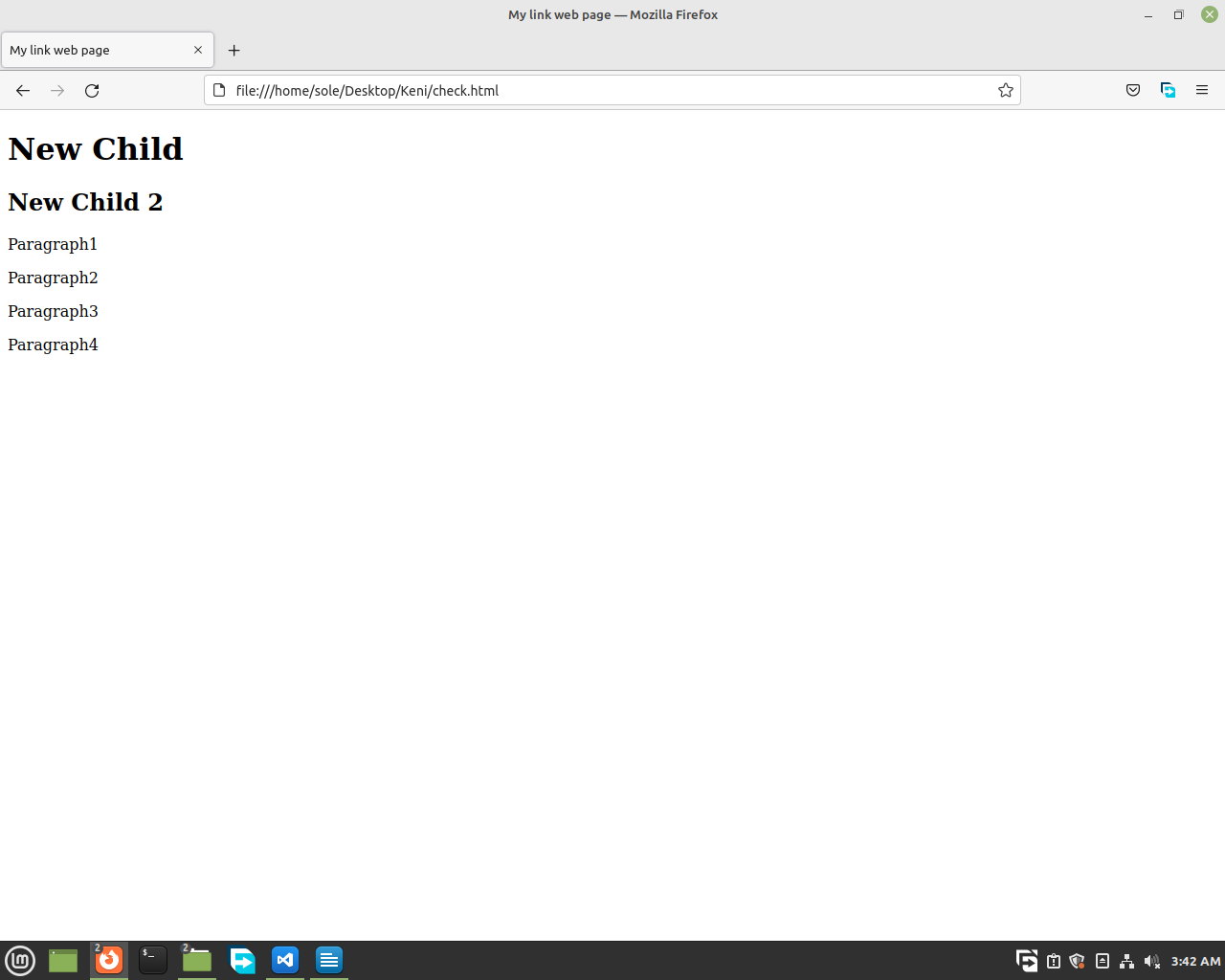
Nothing will change. You might have guessed h2 to change, but the thing that prevents it from changing is the fact that it doesn’t have the class “par”. It selects it if and only
if it has the class “par”. Even if it is the second child, it must have the class name “par”.